How to make a simple library to track any method you call? [Java]
There are many reasons when want to track a method that we call. The common one is to track how much time a method is taking. However, there is a simple way to track time by adding a few lines of code just before the method call and after the method call. But it's not easy when you have hundreds of methods. But having a separate library makes your code cleaner. So without any delay let's see the example program. In the below program, we have a class called FunctionCaller. This is the class responsible to call your given methods and also returns value if any. It has two methods one is for void-methods and one for methods that return something. On the other hand, we have a typical Appium script that launches the application to perform swipe. In the Appium simple program, we are passing our method to the FunctionCaller class that is going to execute your method and returns value if any. As you can see, we have written time tracking logic inside the methods of FunctionCaller class. You can write your own logic too. If you have any scenario where you want to perform any action before calling the method or after calling the method. For now, we are just trying to track the execution time.
import java.net.URL;
import java.time.Duration;
import java.util.concurrent.Callable;
import org.openqa.selenium.Dimension;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.remote.DesiredCapabilities;
import io.appium.java_client.AppiumDriver;
import io.appium.java_client.TouchAction;
import io.appium.java_client.android.AndroidDriver;
import io.appium.java_client.touch.WaitOptions;
import io.appium.java_client.touch.offset.PointOption;
public class TimeTracker {
static AppiumDriver driver;
public static void main(String[] args) throws Exception {
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability("newCommandTimeout", 600);
capabilities.setCapability("launchTimeout", 90000);
capabilities.setCapability("deviceName", "PL2GAR4832302659");
capabilities.setCapability("platformName", "Android");
capabilities.setCapability("rotatable", true);
capabilities.setCapability("appPackage", "com.fsn.nykaa");
capabilities.setCapability("appActivity", "com.fsn.nykaa.SplashScreenActivity");
capabilities.setCapability("app", System.getProperty("user.dir") + "/Crypto.apk");
driver = new AndroidDriver(new URL("http://127.0.0.1:4723/wd/hub"), capabilities);
Thread.sleep(10 * 1000);
System.out.println("Driver Created");
FunctionCaller.callVoidFunction(() -> swipeRight(), "Swipe Right Time Taken");
FunctionCaller.callReturnFunction(()-> swipeLeft(), "Swipe Left Time Taken");
System.out.println("Driver Quit");
driver.quit();
}
private static void swipeRight() {
int startX = 0;
int endX = 0;
int startY = 0;
Dimension size = driver.manage().window().getSize();
startY = (int) (size.height / 2);
startX = (int) (size.width * 0.10);
endX = (int) (size.width * 0.90);
new TouchAction(driver).press(PointOption.point(startX, startY))
.waitAction(WaitOptions.waitOptions(Duration.ofMillis(2000))).moveTo(PointOption.point(endX, startY))
.release().perform();
}
private static boolean swipeLeft() {
try {
int startX = 0;
int endX = 0;
int startY = 0;
Dimension size = driver.manage().window().getSize();
startY = (int) (size.height / 2);
startX = (int) (size.width * 0.90);
endX = (int) (size.width * 0.10);
new TouchAction(driver).press(PointOption.point(startX, startY))
.waitAction(WaitOptions.waitOptions(Duration.ofMillis(2000)))
.moveTo(PointOption.point(endX, startY)).release().perform();
return true;
} catch (Exception e) {
return false;
}
}
}
class FunctionCaller {
public static T callReturnFunction(Callable task, String message) throws Exception {
long startTime = System.currentTimeMillis();
T call = task.call();
String timeTaken = (System.currentTimeMillis() - startTime) + "ms";
System.out.println("Return: " + message + " : " + timeTaken);
return call;
}
public static void callVoidFunction(Runnable aMethod, String message) {
long startTime = System.currentTimeMillis();
aMethod.run();
String timeTaken = (System.currentTimeMillis() - startTime) + "ms";
System.out.println("Void: " + message + " : " + timeTaken);
}
}
Output:
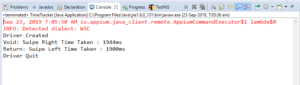