Introduction:
Automation engineers and product managers constantly grapple with the challenge of enhancing app testing efficiency while ensuring comprehensive coverage across various platforms. In this pursuit, Selenium Grid emerges as an indispensable asset, offering a scalable solution that transcends the limitations of traditional testing environments. By facilitating concurrent test execution across multiple machines and browsers, Selenium Grid empowers teams to expedite test cycles, optimize resource utilization, and uncover intricate bugs that might remain undetected in isolated testing scenarios. Moreover, its versatility enables organizations to replicate real-world user scenarios, ensuring that applications deliver a consistent and seamless user experience across diverse environments.
What is Selenium Grid?
Selenium Grid serves as a pivotal component within the Selenium ecosystem, designed explicitly to address the challenges associated with testing in diverse environments. At its core, Selenium Grid functions as a distributed test execution framework, allowing organizations to execute tests concurrently across multiple nodes (machines) and browsers, thereby accelerating test cycles and enhancing test coverage.
How Does it Work?
At a fundamental level, Selenium Grid comprises of two main components: the Hub and Nodes. The synergy between the Hub and Nodes fosters a collaborative testing environment, where the Hub efficiently manages and distributes test execution tasks across multiple Nodes, ensuring seamless coordination and synchronization.
Moreover, this distributed architecture enhances scalability by allowing organizations to add or remove Nodes dynamically based on testing requirements, thereby accommodating fluctuating workloads and optimizing resource allocation. Making it an efficient, scalable, and comprehensive automated testing process to run across diverse environments and configurations.
- The Hub serves as the nerve center of the Selenium Grid architecture, orchestrating the entire testing process. When a test request is initiated, the Hub receives this request and acts as a traffic controller, determining which Node is best suited to execute the test based on predefined criteria such as browser type, version, and operating system. Essentially, the Hub maintains a registry of available Nodes and manages the allocation and distribution of test execution tasks, to ensure optimal utilization and efficient test execution.
- Nodes represent the execution endpoints where the actual tests are executed. Nodes register themselves with the Hub, indicating their availability and capabilities (e.g., browser type, version, platform). Once a test is initiated, the Hub dispatches it to a Node based on the criteria. Each Node operates independently, executing the assigned test scenarios in isolation, and communicates the test results back to the Hub upon completion. By leveraging multiple Nodes, Selenium Grid enables testers to execute tests concurrently across various configurations, browsers, and platforms, thereby enhancing the test coverage and accelerating the testing process.
Benefits of Selenium Grid
Selenium Grid stands as a cornerstone in the domain of automated testing, so
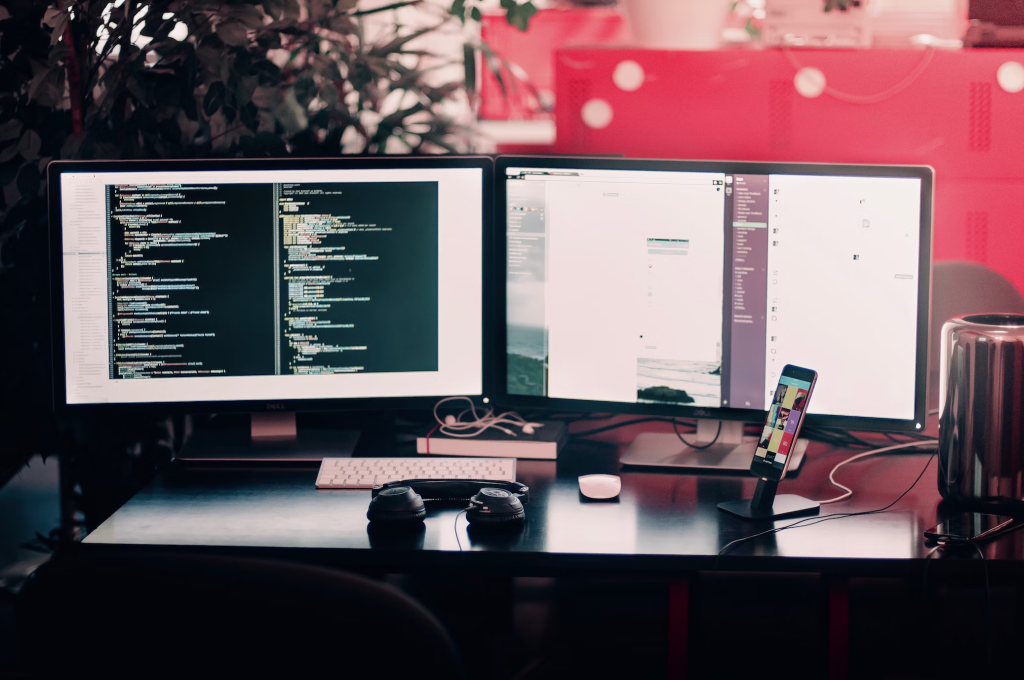
- Enhanced Test Coverage: Selenium Grid empowers organizations to execute tests across a myriad of configurations, including different browsers, versions, and operating systems. This versatility ensures comprehensive test coverage, identifying potential issues across diverse environments and configurations.
- Efficient Resource Utilization: By distributing test execution across multiple nodes, Selenium Grid optimizes resource utilization, enabling organizations to maximize the efficiency of their testing infrastructure. This distributed approach mitigates bottlenecks and ensures seamless test execution, even during peak load periods.
Download a Free Poster That Highlights the Benefits of Selenium Grid
- Consistent User Experience: Selenium Grid facilitates cross-browser testing, enabling organizations to evaluate application compatibility across various browsers such as Chrome, Firefox, Safari, and Edge. This comprehensive testing approach ensures a consistent user experience, irrespective of the browser or platform used by the end-users.
- Browser Compatibility: Selenium Grid empowers testers to identify and rectify browser-specific issues, ensuring optimal functionality across all supported browsers. By leveraging Selenium Grid’s cross-browser testing capabilities, organizations can enhance application compatibility and deliver a seamless user experience across diverse platforms.
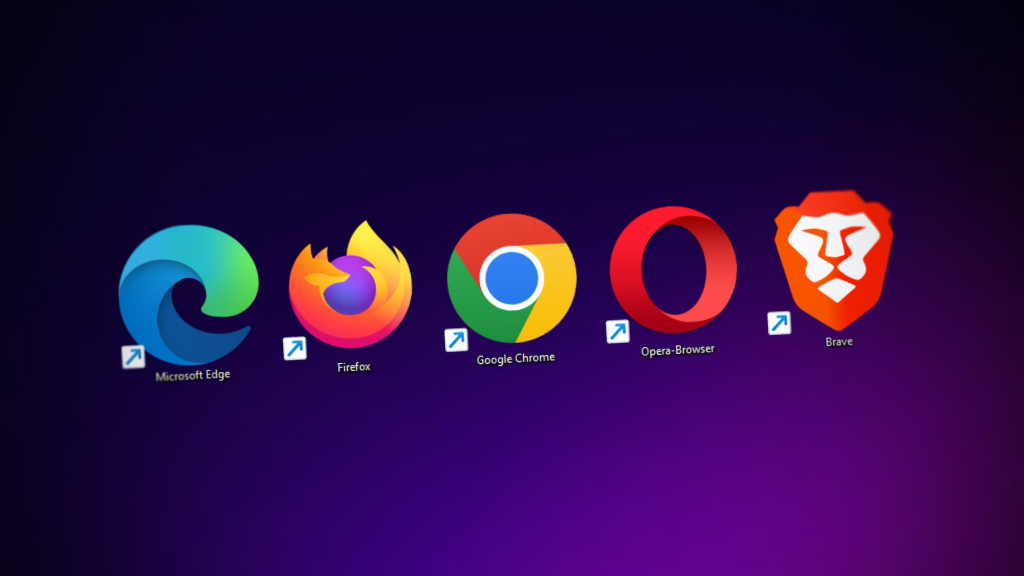
· Reduced Infrastructure Costs: Selenium Grid’s distributed testing capabilities enable organizations to consolidate their testing infrastructure, reducing hardware and maintenance costs associated with maintaining multiple test environments. This cost-effective approach ensures optimal resource allocation, enabling organizations to achieve their testing objectives without incurring exorbitant expenses.
· Faster Time-to-Market: By accelerating test execution and enhancing test coverage, Selenium Grid enables organizations to expedite the application development lifecycle, facilitating faster time-to-market. This competitive advantage empowers organizations to capitalize on market opportunities and gain a competitive edge in today’s rapidly evolving digital landscape.
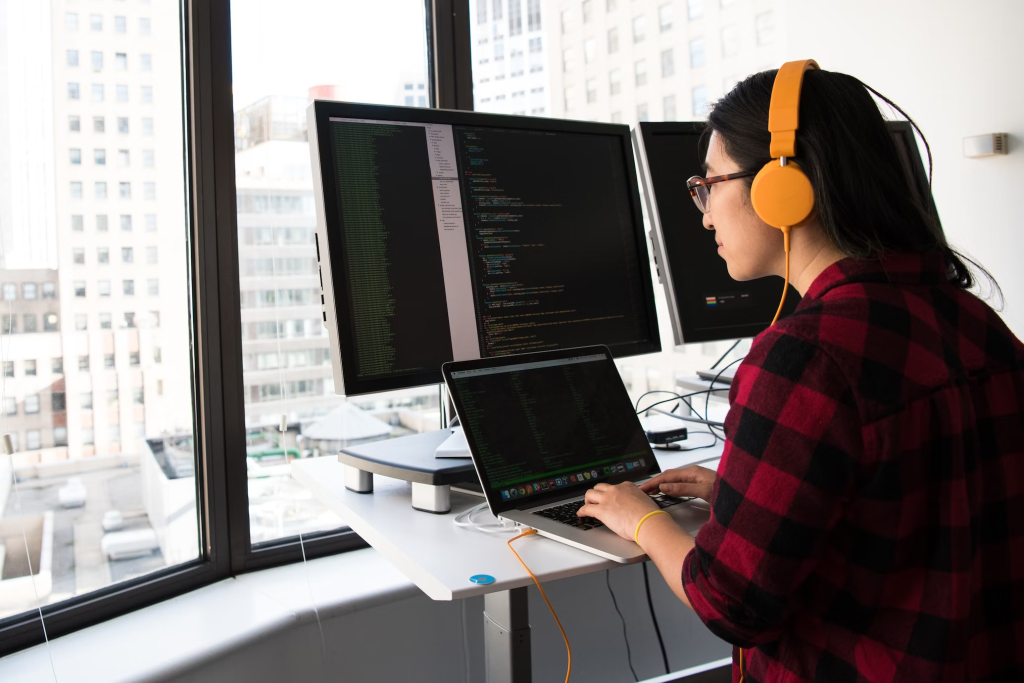
- Accelerated Test Cycles: Selenium Grid facilitates parallel test execution, enabling organizations to execute multiple test cases simultaneously across different nodes. This parallel execution capability accelerates test cycles, enabling organizations to expedite the release cycles and deliver high-quality applications within stipulated timelines.
- Enhanced Productivity: By leveraging Selenium Grid’s parallel execution capabilities, organizations can enhance tester productivity and optimize resource utilization. This efficient testing approach minimizes idle time and ensures continuous test execution, enabling organizations to achieve their testing objectives with utmost precision and efficiency.
Sample Automation Script: Opening and Closing Pcloudy Website
To demonstrate the capabilities of Selenium Grid, let’s create a simple automation script using Selenium WebDriver and Java to open the Pcloudy website and close it.
Prerequisites:
- Java Development Kit (JDK)
- Selenium WebDriver
WebDriver-compatible browser drivers (e.g., ChromeDriver)
Sample Script:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.URL;
public class SeleniumGridDemo {
public static void main(String[] args) {
try {
// Define the desired capabilities
DesiredCapabilities capabilities = DesiredCapabilities.chrome();
// Specify the URL of the Selenium Grid Hub
URL hubUrl = new URL(“http://<hub_ip_address>:4444/wd/hub”); // Replace <hub_ip_address> with the actual IP address
// Create a new WebDriver instance pointing to the Selenium Grid Hub
WebDriver driver = new RemoteWebDriver(hubUrl, capabilities);
// Navigate to the Pcloudy website
driver.get(“https://www.pcloudy.com/”);
// Print the title of the webpage
System.out.println(“Title of the webpage: ” + driver.getTitle());
// Close the browser
driver.quit();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Code Walkthrough
Importing Essential Libraries:
In programming, libraries are pre-written sets of code that offer functionalities to simplify tasks. Here, we’re importing the necessary tools to interact with web browsers and Selenium Grid.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.URL;
Configuring Browser and Grid Settings:
Before executing tests, we need to specify which browser and Selenium Grid Hub we’ll be using.
DesiredCapabilities capabilities = DesiredCapabilities.chrome();
URL hubUrl = new URL(“http://<hub_ip_address>:4444/wd/hub”);
- chrome(): sets our test environment to use the Chrome browser. Desired capabilities allow us to define specific browser configurations like version, platform, etc.
URL(“http://<hub_ip_address>:4444/wd/hub”): establishes a connection to the Selenium Grid Hub. Replace <hub_ip_address> with the actual IP address where your Selenium Grid Hub is running.
3. Initializing WebDriver
WebDriver is like our digital driver, driving the browser to perform actions based on our instructions.
WebDriver driver = new RemoteWebDriver(hubUrl, capabilities);
new RemoteWebDriver(hubUrl, capabilities): initializes a new browser session through the Selenium Grid Hub using the specified capabilities (Chrome browser in our case).
4. Navigating to a Website:
With the browser up and running, we instruct WebDriver to navigate to a specific URL, simulating user behavior.
driver.get(“https://www.pcloudy.com/”);
driver.get(“https://www.pcloudy.com/”): This command directs the WebDriver to open the Pcloudy website in the Chrome browser.
5.Retrieving Website Information:
After accessing the website, we extract and display specific information, such as the webpage title.
System.out.println(“Title of the webpage: ” + driver.getTitle());
- driver.getTitle(): Retrieves the title of the current webpage opened by the WebDriver instance.
System.out.println(): Prints the retrieved webpage title to the console for verification and logging purposes.
Terminating the WebDriver Session:
Finally, once our test tasks are complete, we gracefully close the browser session.
driver.quit();
- driver.quit(): This command terminates the browser session, freeing up system resources and concluding our automated test scenario.
This automation script essentially guides a series of actions using Selenium WebDriver, letting us automate our interactions with web browsers. Using the power of Selenium Grid, we’re spreading out and running these tests on different setups, browsers, and systems. It’s like having multiple hands at work, making our automation tasks smoother, faster, and more flexible.
Conclusion:
Selenium Grid emerges as a game-changer in the realm of automated testing, offering unparalleled benefits that enhance efficiency, scalability, and reliability. By leveraging Selenium Grid’s robust capabilities, organizations can achieve faster test execution, improved test coverage, and cost-effective test automation solutions. Embracing Selenium Grid empowers organizations to navigate the complexities of modern application development, ensuring optimal quality, performance, and user satisfaction across diverse platforms and configurations.