Introduction:
Selenium with Python programming language is a powerful combination for web automation, which helps both testers and developers to interact with web applications efficiently.
In web automation with Selenium and Python, it’s not only about simple commands. It’s about using special action words that work like secret tools for smooth interactions in Advanced web automation. While the fundamental Selenium commands open doors, these advanced actions are like superhero movies that reveal the hidden wonders within web applications.
So, why do we need these advanced actions, you ask?
It’s like unlocking superpowers to perform tasks that go beyond the ordinary, making the entire web automation journey more remarkable!
In this blog, we’ll explore the need for advanced actions, discuss powerful techniques, provide code examples using the https://qa-practice.netlify.app/bugs-form.html website, and discuss the benefits of employing these advanced Selenium functionalities. We will understand how using these techniques can help us perform advanced web automation.
An Autonomous Bot to Test your Apps
Why Advanced Actions?
Basic Selenium commands are perfect for simple interactions like clicking buttons and entering text. However, real-world web applications often require more sophisticated automation. Advanced actions come into play when handling dynamic content, waiting for specific conditions, or dealing with complex user interactions. Let’s dive into seven advanced Selenium actions with practical examples.
Advanced Actions with Code Examples:
Handling Dynamic Elements:
Definition:
Locating and interacting with elements that appear or disappear dynamically on a webpage.
Scenario:
Imagine a form that dynamically adds new input fields based on user selections. Using basic commands might result in locating non-existent elements. Instead, employ advanced actions to wait for the appearance of dynamic elements.
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, “dynamicElementID”))
)
element.click()
Working with Frames:
Definition:
Switching between frames within a webpage to perform actions in specific contexts.Refer the link here for an Iframe example: https://qa-practice.netlify.app/iframe
Scenario:
You encounter a webpage with multiple frames, and your script needs to interact with elements inside these frames. Basic Selenium commands won’t work outside the default content. Advanced actions allow you to switch context seamlessly.
driver.switch_to.frame(“frameName”)
# Perform actions inside the frame
driver.switch_to.default_content()
Mouse Actions - Hover:
Definition:
Simulating mouse hovering to interact with elements that are only visible on hover.
Scenario:
You have a navigation menu where additional options appear only when hovered over. Basic commands might miss these elements. Advanced actions, like mouse hovering, ensure accurate interactions.
from selenium.webdriver.common.action_chains import ActionChains
element_to_hover_over = driver.find_element(By.ID, “hoverElementId”)
hover = ActionChains(driver).move_to_element(element_to_hover_over)
hover.perform()
Handling Alerts:
Definition:
Managing pop-up alerts and confirming or dismissing them.
In the Selenium world, “Alert Handling” involves managing those sudden pop-up messages that interrupt your automated website tests. These pop-ups might throw questions at you, like confirming a critical action such as clicking a button, or they could demand your input.
Alert Types
Confirmation Alerts:
Imagine these as virtual sticky notes that pop up and ask, “Are you really sure you want to do this?” responding with either “OK” to proceed or “Cancel”. It’s like a virtual double-check before committing to an important action.
Prompt Alerts:
These pop-ups ask you to type in some information. For instance, you might need to enter a username or give feedback.
Simple Alerts:
These are basic pop-ups just showing a message, like a notification.
Handling alerts in Selenium is important for testers because it lets your automated tests interact with these pop-ups in the same way a person would. So, you can confirm actions, provide input, or handle notifications during your automated testing. Below is an example screenshot on an Alert:
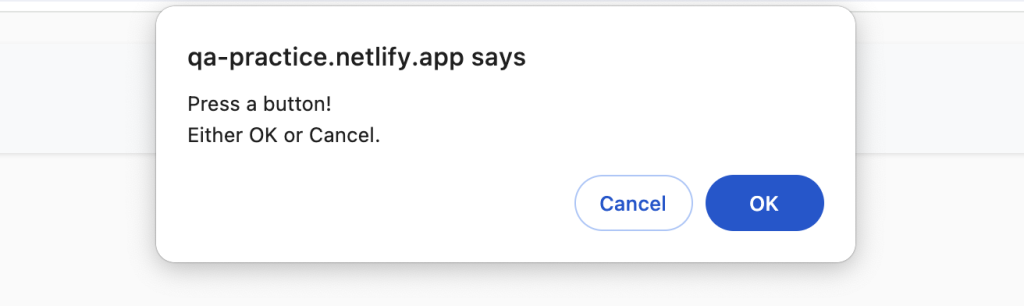
Scenario:
An alert prompts the user for confirmation before submitting a form. Basic Selenium commands won’t handle alerts. Advanced actions are essential for interacting with pop-ups.
alert = driver.switch_to.alert
# Accept the alert
alert.accept()
# Reject the alert
alert.dismiss()
# Send text to the alert prompt
alert.send_keys(“Your Text Here”)
Keyboard Actions
In Selenium, we can use keyboard actions to perform special key-related tasks. This includes pressing keys, releasing them, and combining multiple keys for specific actions.
When you are automating tests with Selenium, sometimes you need to simulate keyboard actions. This means making your program press keys, release them, or even press a combination of keys together.
For example:
- Pressing the “Enter” key.
- Holding down the “Shift” key while pressing another key.
- Releasing a key that you previously pressed.
These keyboard actions can be useful to deal with keyboard input.
from selenium.webdriver.common.keys import Keys
# Sending keys to an input field
text_field = driver.find_element_by_id(“text-field-id”)
text_field.send_keys(“Hello, World!”)
# Combining keys (e.g., Ctrl+A to select all text)
text_field.send_keys(Keys.CONTROL + “a”)
Executing JavaScript
Definition:
Running custom JavaScript code to manipulate the DOM or perform specific actions.
Scenario:
You encounter a situation where a simple click doesn’t trigger the expected behavior. Advanced actions, like executing JavaScript, offer a more flexible approach.
driver.execute_script(“window.scrollTo(0, document.body.scrollHeight);”)
We can also use it to fetch inner text like below:
element = driver.find_element_by_id(“yourElementId”)
inner_text = driver.execute_script(“return arguments[0].innerText;”, element)
Advanced Waits
Definition:
Implementing custom wait conditions to ensure elements are clickable, visible, etc., before interacting with them.
Scenario:
Your automation script needs to click a button that appears after a delay or once a certain condition is met. Basic commands might fail in this scenario. Advanced waits ensure synchronisation with the webpage.
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EXC
element = WebDriverWait(driver, 10).until(
EXC.element_to_be_clickable((By.ID, “someElementID”))
)
element.click()
Browser Navigation
Definition:
Navigating through the browser history – going back, forward, or refreshing the page.
Scenario:
After you send a form, go back to the earlier page to check how the form has affected things.. Basic commands lack this capability. Advanced navigation actions help you traverse the browser history.
driver.back()
driver.forward()
driver.refresh()
Conclusion:
Embracing Selenium Python frameworks is a game-changer for advanced web automation. These frameworks provide the structure, scalability, and efficiency needed to navigate the complexities of modern web applications. Whether you opt for Robot Framework’s keyword-driven simplicity or Behave’s BDD approach, integrating these frameworks into your Selenium workflow empowers you to build and maintain robust automation scripts. Elevate your web testing capabilities and embark on a journey towards more efficient and reliable automation with Selenium and Python frameworks. Happy testing!