Introduction
In the agile environment, developers need to run relevant cross-browser tests to push any front-end changes quickly. While small projects use manual testing, larger projects need automation because of the increasing number of web browsers. How is this done using Selenium WebDriver and Python? Selenium is one of the most popular open-source web automation testing tools available today. Python on the other hand is picking up steam since the last decade and is highly favored among testers for automation testing, security testing, extracting data from the web, etc. Let us look at a step-by-step guide highlighting how to perform Web automation testing using Selenium WebDriver and Python and try to answer why Selenium and Python make a formidable pact? Can we use Python in Selenium WebDriver? etc., as we move ahead in this article.
Selenium Webdriver with Python: Overview
We use Selenium for test automation because Selenium is a flexible automation testing platform. It supports many programming languages like C#, Ruby, Perl, Java, Python, etc. It also accommodates different operating systems and web browsers. Mozilla Firefox is the default web browser for Selenium WebDriver. It integrates well with other test automation tools for test case management and test report generation.
Can we use Python in Selenium WebDriver? Python is a High-Level Object Oriented Language used for scripting. It is less complicated and has less syntax as compared to other programming languages. Some of the commands used in Python are- Else if, Else, etc., which are easily understandable English words. Although Selenium supports most of the programming languages, the reason why tech teams prefers use Selenium WebDriver with Python for testing is because of the following pointers:
– Python is simple and easy to work on,
– It is open-source,
– It is understood by most of the developers,
– It comes with a broad community support,
– There are many Python Test automation frameworks available,
– It requires less code to complete a task;
– It is easy to perform parallel testing with Python, and so on.
Web Automation using Selenium WebDriver with Python: Prerequisites
Now that we have an idea about Selenium WebDriver and Python, let us dig deeper to understand how to use Selenium WebDriver with Python. Let us get started with setting up Selenium WebDriver with Python on Windows, step-by-step.
1. Download and install Python for Windows. Ignore if already installed.
2. Install Selenium on Python Environment through the preferred installer program (PIP). It is a prerequisite for installing and managing any Python package. To download PIP on windows, simply click on this link and save it on your system.
Now execute, https://bootstrap.pypa.io/get-pip.py command on the terminal to
install it on the saved PIP file. You can confirm if the PIP is installed successfully on your
machine, by running the following command: pip –version.
3. Install the PyTest Framework by executing pip install –U pytest command, and to verify if the installation was successful, run pytest –version command. This is how it should look like :

4. To Install Selenium framework, if not installed, execute this command:
pip install -U selenium
To retrieve which version of Python is installed, execute the following command:
python -c “import selenium; print(selenium.__version.
To verify if everything is working well, use the following code:
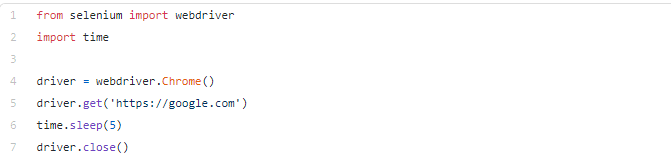
5. Now, if the test is to be performed on the chrome browser, you would need drivers to be able to interact with the Chrome Browser. You can choose other browsers of your choice. In the case of Chrome, you can download a compatible Chrome WebDriver as per the version of Chrome on your system here .
You should note that downloading the packages and drivers is enough for testing locally with Selenium. But if you plan to set up Selenium remotely, you need to install Selenium Server from its download page. It is Java-based and requires JRE 1.6 or above to install it on your server.
Running automated tests using Selenium with Python
Once the prerequisites are taken care of, you can straight away start your first Selenium with Python test.
- Import WebDriver (to connect you to browser instance) and Keys Class (to emulate keyboard keys strokes) from Selenium using the below command:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
- Create Chrome Instance with driver path downloaded from the browser website. Now, assuming that the driver and the Python script to be executed are in the same directory. The following command can be used to open the Chrome instance locally on your machine. You can perform tests and use the .close() method to end the connection to the browser.
driver = webdriver.Chrome('./chromedriver')
- Now, the .get() method is used to load the website. It lets the website render completely before moving to the next step.
driver.get("https://www.python.org")
- Next, use the .title attribute [print(driver.title)] to access the textual title of the webpage. This will print the title of the page as; Welcome to Python.org
- Let us now submit a query in the search bar by selecting an element from HTML DOM> enter value> submit the form by pressing the emulated Enter key. How do you select elements? – use CSS Class, ID, tag name, name attribute (whose DOM Element name at source is ‘q’). To select element, use the .find_element_by_name() method.
search_bar = driver.find_element_by_name("q")
- After this step, use the .clear() method to clear the contents of the DOM element, Use the .send_keys() method to enter a string as its value and press emulated return key using Keys.RETURN
search_bar.clear()
search_bar.send_keys("getting started with python")
search_bar.send_keys(Keys.RETURN).
These actions naturally change the URL with search results in the window. Use the print(driver.current_url) command to confirm the current URL.
You will see the following string:
‘https://www.python.org/search/?q=getting+started+with+python&submit=‘
Close the session and disconnect from the browser using the .close() method.
This was an example of using the Selenium WebDriver to showcase how to run an automated test using Selenium with Python.
Here is the first test on Selenium WebDriver with Python. Save the file selenium_test.py, and to run the test, run python selenium_test.py.
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome('./chromedriver')
driver.get("https://www.python.org")
print(driver.title)
search_bar = driver.find_element_by_name("q")
search_bar.clear()
search_bar.send_keys("getting started with python")
search_bar.send_keys(Keys.RETURN)
print(driver.current_url)
driver.close()
Af
- port WebDriver (to connect you to browser instance) and Keys Class (to emulate keyboard keys strokes) from Selenium using the below command:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
- Create Chrome Instance with driver path downloaded from the browser website. Now, assuming that the driver and the Python script to be executed are in the same directory. The following command can be used to open the Chrome instance locally on your machine. You can perform tests and use the .close() method to end the connection to the browser.
driver = webdriver.Chrome('./chromedriver')
- Now, the .get() method is used to load the website. It lets the website render completely before moving to the next step.
driver.get("https://www.python.org")
- Next, use the .title attribute [print(driver.title)] to access the textual title of the webpage. This will print the title of the page as; Welcome to Python.org
- Let us now submit a query in the search bar by selecting an element from HTML DOM> enter value> submit the form by pressing the emulated Enter key. How do you select elements? – use CSS Class, ID, tag name, name attribute (whose DOM Element name at source is ‘q’). To select element, use the .find_element_by_name() method.
search_bar = driver.find_element_by_name("q")
- After this step, use the .clear() method to clear the contents of the DOM element, Use the .send_keys() method to enter a string as its value and press emulated return key using Keys.RETURN
ter the successful running of the test in Selenium WebDriver with Python, let us also take a quick sneak into:
How to interact with various DOM elements? Following is the HTML of the Search bar:
<input id="id-search-field" name="q" type="search" role="textbox"
class="search-field" placeholder="Search" value="" tabindex="1">
Here, the .find_element_by_name() method is used to search attribute name in the HTML Tag.
You can also use other methods like,
.find_element_by_id(“id-search-field”) for CSS ID,
.find_element_by_xpath(“//input[@id=’id-search-field’]”) for DOM Path
.find_element_by_class_name(“search-field”), for CSS Class
How to navigate Windows and Frames?
-When you work with an application, you deal with multiple windows and frames, commonly while logging into your social accounts or uploading files. Using the .switch_to_window() method of the driver will help you change the active window and explore different actions on the new one using the command driver.switch_to_window(‘window_name’)
-For viewing all the window handles, run print(driver.window_handles). Windows handle is used in case the target attribute does not store the value. It individually identifies all open windows in the driver.
– To shift to frame within a window, use .switch_to_frame() method and to switch back to the window again after completing relevant tasks, run: driver.switch_to_default_content()
The actions performed in this section only change the driver’s status and do not display anything in the result. Hence, we call the methods because values are not stored in the variables.
Handle Idle Time During a test
Selenium involves implicit and explicit waits. In the case of Explicit wait, the driver waits for a specific action to complete and in implicit wait, the driver waits for a particular time duration. Using WebDriverWait() function will help the WebDriver to wait for a specific time, say five seconds. Then to test for new element to load, use: .presence_of_element_located() method (belonging to expected_conditions class); Use By.ID.
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
try:
element = WebDriverWait(driver, 5).until(
EC.presence_of_element_located((By.ID, "id-of-new-element"))
)
finally:
driver.quit()
Use the .implicitly_wait() method to offer a specific number of seconds for the driver to wait.
driver.implicitly_wait(5)
element = driver.find_element_by_id("id-of-new-element")
Integrate Selenium with Python Unit Tests
For integrating Selenium tests into Python Unit tests, use the following module in Python:
import unittest
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
class ChromeSearch(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome('./chromedriver')
def test_search_in_python_org(self):
driver = self.driver
driver.get("https://www.python.org")
self.assertIn("Python", driver.title)
elem = driver.find_element_by_name("q")
elem.send_keys("getting started with python")
elem.send_keys(Keys.RETURN)
assert "https://www.python.org/search/?q=getting+started+with+python&submit=" == driver.current_url
def tearDown(self):
self.driver.close()
if __name__ == "__main__":
unittest.main()
In the above Selenium WebDriver Python example, while starting the unit test class use the.Chrome() method first to set up the driver object. The same text is put in the search bar with every test, and changes in the URL (output vs. initial) are observed and compared. Also, the different tests can be written for different browsers re-using the same functionality.
The combination of Selenium WebDriver with Python offers numerous benefits for web automation testing. Here are some key advantages:
1) Easy to learn and use: Python is known for its simplicity and readability. Its intuitive syntax and English-like keywords make it easier for beginners to grasp the language and write automation scripts. The straightforward nature of Python allows testers to quickly learn and start using Selenium WebDriver for web automation without facing steep learning curves.
2) Extensive community support: Python has a large and vibrant community of developers. This community actively contributes to the development of libraries, frameworks, and resources specifically tailored for Selenium automation. This abundance of community support ensures that there are ample resources available to help you solve problems, find answers to questions, and access ready-to-use code snippets and examples.
3) Wide range of libraries and frameworks: Python boasts an extensive ecosystem of libraries and frameworks that can be utilized in conjunction with Selenium WebDriver for web automation testing. These libraries and frameworks provide additional functionalities, such as handling database connections, generating test reports, performing data manipulation, and interacting with APIs. With Python, you can easily integrate other tools and technologies into your automation workflow, enhancing the capabilities of your tests and streamlining your testing process.
4) Cross-platform compatibility: Selenium WebDriver with Python is compatible with various operating systems, including Windows, macOS, and Linux. This cross-platform compatibility allows testers to write automation scripts on one platform and seamlessly execute them on different operating systems without requiring major modifications. This flexibility saves time and effort, as you can test your web applications across different environments and ensure their compatibility with diverse user setups.
5) Integration capabilities: Python’s versatility extends beyond Selenium WebDriver. It can be effortlessly integrated with other tools, technologies, and frameworks commonly used in the software development and testing landscape. For instance, you can integrate Python with test management systems, continuous integration tools, logging frameworks, and reporting tools to enhance your automation workflow. This integration capability enables you to build a comprehensive and customized automation ecosystem tailored to your specific project requirements.
Conclusion
It is now known to everyone that the Selenium WebDriver is the most preferred tool for web automation. It is an open-source tool and, most importantly, supports widely used programming languages like Python, Java, C#, etc. There are various methods of automating the cross-browser testing process using Selenium WebDriver with Python programming language. We have to use compatible browser drivers for respective browsers on which we want to perform automation testing so that interaction with the web browsers is possible. Also, using a cloud-based Selenium Grid like pCloudy helps in performing parallel test execution. To answer how we can use Python in Selenium WebDriver, we have explained it with the help of different Selenium WebDriver Python examples.
More than automation, what matters is, how strongly you have designed your test cases to cover all possible scenarios. The importance of testing is experienced when we catch the errors during the testing phase before they transform into a customer complaint.
