With many apps being developed at a rapid pace, testing and releasing the apps is starting to become a challenge. However, with the use of various rapid automation techniques and automation tools like Selenium, testing teams are able to test early, resolve issues and release apps faster. Selenium automation has proven to be an excellent tool to automate test cases for faster app testing. Selenium and Python make a formidable pact to accelerate testing of web apps. The Selenium Python Bindings helps provide a simple API to write functional/acceptance tests for the Selenium WebDriver. Let us dig a little deeper to learn more about Selenium, Python and language binding to understand the concept a little better.
What is Selenium?
Selenium is a popular open source automation framework that is used to automate your web app testing. It is used to validate web applications across various browsers like IE, Firefox, Chrome, etc. The framework even supports various programming languages like C#, Python, Java, etc. Selenium is not just a single tool but a test suite that comprises various tools that ease up the automation process of testing web applications. There is a dire need to scale up testing your web application and website, and Selenium fulfills this need through selenium webdriver python bindings.
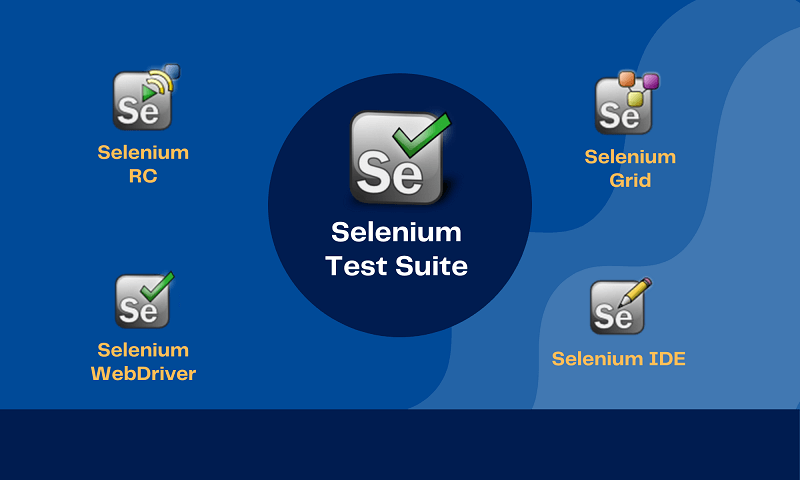
Selenium Remote Control (RC)
Selenium Remote Control or Selenium RC, was introduced to tackle the problem of installing the application and the Selenium Core to perform the testing activities. Selenium RC was created to act as a HTTP proxy to overcome the tedious task of installing the entire application that needs to be tested and the Selenium core on the local machine. Now with the help of Selenium RC users can use various programming languages to automate their web app testing efforts. Selenium RC is also called Selenium
Selenium IDE
Selenium Integrated Development Environment (IDE), is a simple framework that is part of the Selenium Test Suite. It is a firefox extension that can be used to automate the browser through the record and playback feature. You can easily install and use this plugin for building some basic test cases. For more complex and complicated test cases it is advisable to use Selenium RC or WebDriver.
Selenium Grid
Selenium Grid was developed by Patrick Lightbody to minimize the number of test executions in app automation. Selenium Grid can be used with Selenium RC to execute tests across different machines and browsers parallely at the same time. Selenium Grid is great for parallel test executions.
Selenium WebDriver
Selenium WebDriver is another framework within the Selenium test suite to automate different browser actions across different browsers. Unlike the Selenium RC or Selenium IDE, Selenium WebDriver uses a modern and stable approach to automate the browser actions while performing testing. It is also not restricted to any particular programming language and supports Java, C#, PHP, Python, Perl, and Ruby. It controls the browser by directly connecting with it from the system itself.
Download a free Poster explaining the various tools in the Selenium Test Suite
What is Python?
Python is a high-level programming language with diverse functions and dynamic semantics. It is used for various tasks such as website building, software development, data analysis and automation scripting. Python is a general purpose programming language that can be used for multiple tasks and solving problems. It is the most widely used programming language because of its beginner friendliness, easy to learn aspects. Python is used as the go-to programming language for writing automation scripts in the QA space. While there are a lot of ways that Python can be used in app testing, we will specifically look at Python binding for Selenium.
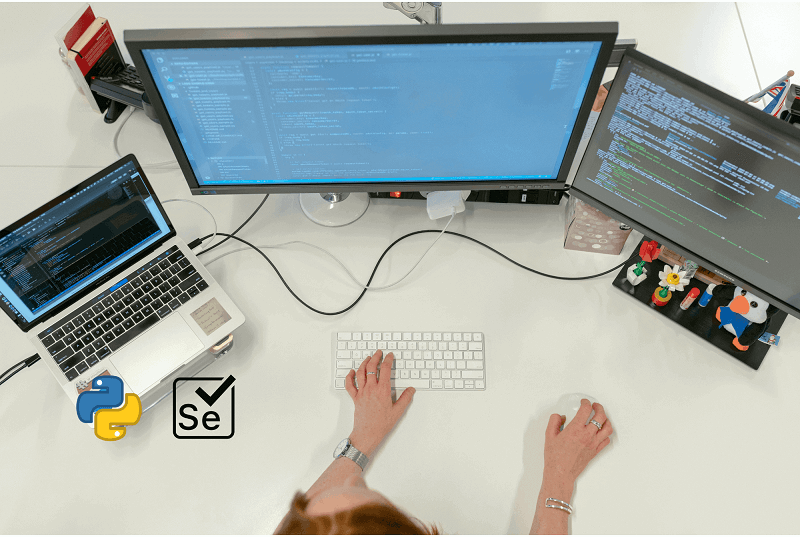
What are language bindings with Selenium?
Before we jump straight into Selenium python bindings, let us first understand the concept of language bindings with selenium. Selenium WebDrivers are used to automate browser actions directly from your system. Now, since Selenium supports all programming languages such as C#, Python, Java, etc. there is no one specific language that a user will have to use when writing an automation script for Selenium WebDriver. At the same time the code provided to you by Selenium to you as a developer is called the Selenium language binding. The bindings provided look similar to the language working in to make it easy to write the scripts. For example, if the python bindings will look similar to python, while the Java binding will look normal to the Java code. Language bindings help developers with an ease of working with selenium to automate the tests.
What are Selenium-Python Bindings?
Selenium Python bindings provide an easy to use API to write functional acceptance tests using Selenium WebDriver. The Selenium Python API allows users to access various functionalities of the Selenium WebDriver in an intuitive way. The Selenium Python Bindings also allows users to easily access various Selenium WebDrivers like Chrome, Firefox, IE, etc. The Selenium-Python Binding allows users to easily perform functions on Python to automate the browser actions for testing.
Tools required for Selenium-Python Binding
- Python
- Python Binding from Selenium
- Selenium Package
- Browser Drivers
Installation
You can install the Python bindings for selenium by getting the selenium package from pip. Python 3 has the pip available in its standard library. You can simply install it from there using the command below.
pip install selenium
We will need to use virtualenv to create isolated Python environments. You can even download and install the Selenium-Python Binding manually from the PyPI Selenium Package page. If you are running a Windows machine, you can directly install Python 3 from python.org and run it from the command line. For those of you who want to install from Git you can simply clone the official repository. You can access the Python code from the /py directory.
Drivers
You will need to have the browser drivers for Selenium to interact with the browsers. You will also need to ensure that the drivers are in your PATH. for e.g. you can place them in /usr/bin or /usr/personal/bin. Not including the drivers in the PATH will throw out a error as shown below –
selenium.common.exceptions.WebDriverException: Message: ‘geckodriver’ executable needs to be in PATH.
Here is a list of the most popular browser drivers.
Running Selenium Server
For those of you who want to use the remote WebDriver. Running the selenium server becomes an absolute necessity. Selenium Server is a Java Program. It is recommended to have Java Runtime Environment (JRE) 1.6 or newer installed on your computer to run the Selenium server. Once you have a java command in your PATH (environment), you can use the command below.
java -jar selenium-server-standalone-2.x.x.jar
Please note that you will need to replace the 2.x.x. with the actual Selenium version on your system accordingly.
Running Selenium Python Bindings
Once you have installed the Selenium Python bindings, you can start using it on Python.
Here is an example –
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
driver = webdriver.chrome()
driver.get(“http://www.pcloudy.com/”)
assert “pCloudy” in driver.title
elem = driver.find_element_by_name(“q”)
elem.clear()
elem.send_keys(“testing”)
elem.send_keys(Keys.RETURN)
assert “No results found.” not in driver.page_source
driver.close()
You can save the script into a file. For example – pcloudy_search.py and run it using –
python pcloudy_search.py
Code Walkthrough:
We are first importing the selenium webdriver which contains the browsers drivers like chrome, firefox, IE, etc. We are also importing the KEYS class which provides the keys like Return, F1, Alt, etc. on the Keyboard. Next we are creating an instance for the Chrome WebDriver. The driver.get method will navigate to the pCloudy page provided in the URL. The WebDriver will wait for the page to fully load before returning control to the script. Next we are confirming if the page title has the word “pCloudy” in it. We’re using the find_element_by_name method to look for elements using the name attribute.
The WebDriver also provides various methods to find elements, you can read more about it in the Locating Elements chapter of the Selenium Python Binding documentation. Next we are sending the keys in the control, this is similar to entering the keys using the keyboard. We’re also clearing out any pre-populated text in the input field (E.g. “Search”) to avoid it from affecting the search results. You can even send special keys using Keys class imported from selenium.webdriver.common.keys. After the submission of the page, we should get a result if the control detects any. We have also made an assertion to ensure that some results are found. We are calling the close method in the end to close the browser. We can also use the quit method to exit the entire browser. And in case only one tab is open on the browser the close method will exit the browser entirely by default.
Advantages of Selenium Python Bindings:
Selenium Python bindings offer numerous advantages for test automation, making it a popular choice among developers and testers. Here are some key benefits of using Selenium Python bindings:
Easy Integration with Python: Selenium Python bindings provide seamless integration with Python, a widely used programming language known for its simplicity and versatility. Python’s intuitive syntax and extensive libraries make it easier to write and maintain automation scripts, allowing testers to leverage Python’s rich ecosystem for various testing needs.
Wide Range of Supported Browsers: Selenium Python bindings support a wide range of browsers, including popular options like Chrome, Firefox, Safari, and Internet Explorer. This broad browser compatibility ensures that your automated tests can run smoothly across different browsers, enabling comprehensive browser compatibility testing for your web applications.
Utilizing Python’s Ecosystem: By using Selenium Python bindings, you can leverage the vast ecosystem of Python libraries and tools. Python offers a wide range of packages and modules for tasks such as data manipulation, file handling, test reporting, and logging, allowing you to enhance your test automation efforts with additional functionalities and capabilities.
Efficient and Effective Test Automation: Selenium Python bindings enable efficient and effective test automation. With Python’s concise syntax and Selenium’s powerful capabilities, testers can quickly create robust and maintainable automation scripts. This combination allows for faster test script development and execution, leading to shorter testing cycles and faster feedback on the quality of the software under test.
Cross-platform Compatibility: Python itself is a cross-platform programming language, and Selenium Python bindings inherit this cross-platform compatibility. You can develop and execute your automation scripts on various operating systems, including Windows, macOS, and Linux, without major modifications. This flexibility ensures that your tests can be performed on different environments, increasing the coverage of your test scenarios.
Best Practices for Using Selenium Python Bindings:
When working with Selenium Python bindings, it’s important to follow best practices to ensure maintainable and reliable test automation. Here are some tips and recommendations for maximizing the effectiveness of your Selenium Python automation scripts:
Writing Maintainable Automation Scripts:
Follow the principles of clean code and maintainable automation, such as using descriptive and meaningful variable and method names.
Utilize functions, classes, and modules to organize and structure your code in a modular and reusable manner.
Implement a consistent coding style, adhere to PEP 8 guidelines, and use comments to enhance code readability.
Handling Common Automation Challenges:
Use explicit waits and expected conditions to handle dynamic elements and synchronization issues, ensuring that your automation scripts interact with the web application at the right time.
Implement robust error handling and exception handling mechanisms to gracefully handle unexpected pop-ups, alerts, or error scenarios encountered during test execution.
Employ techniques like Page Object Model (POM) to enhance test maintenance and reusability by separating the page elements and their related actions from the test scripts.
Structuring Test Code and Organizing Test Suites:
Organize your test code into logical modules or packages based on the application features or test scenarios.
Implement a clear test hierarchy and structure, using test suites or test runners to group related test cases and manage test execution efficiently.
Leverage Python test frameworks like pytest or unittest to take advantage of built-in features such as test discovery, test fixtures, and test reporting.
Implementing Efficient Test Data Management:
Use data-driven testing techniques to separate test data from the test logic, allowing for easy maintenance and scalability of your test suite.
Store test data in external files (e.g., CSV, JSON, Excel) or databases and design your automation scripts to read the data dynamically during test execution.
Utilize Python libraries for data manipulation and generation to create realistic and comprehensive test scenarios.
By following these best practices, you can enhance the effectiveness of your Selenium Python automation efforts, leading to more reliable and maintainable test scripts, faster testing cycles, and improved software quality.
Advanced Techniques and Features with Selenium Python Bindings
Selenium Python bindings provide a wide range of advanced techniques and features that can enhance your test automation efforts. These capabilities go beyond basic browser interaction and allow you to handle complex scenarios and perform advanced interactions. Here are some advanced techniques and features you can explore with Selenium Python bindings:
Handling iframes: Iframes (Inline Frames) are commonly used to embed content from another source within a web page. Selenium Python bindings offer methods to switch to and interact with elements inside iframes. You can use the switch_to.frame() method to switch the focus to the desired iframe, perform actions within it, and then switch back to the default content.
Working with multiple windows or tabs: Selenium Python bindings provide functions to handle scenarios where your web application opens multiple browser windows or tabs. You can use the window_handles property to retrieve a list of window handles, switch between windows using the switch_to.window() method, and perform operations on each window independently.
Performing advanced interactions: Selenium Python bindings allow you to perform advanced interactions such as drag and drop, mouse hover, double-click, right-click, and keyboard actions. You can use the ActionChains class to chain multiple actions together and perform complex interactions on web elements.
Mobile app testing: Selenium Python bindings also offer capabilities for mobile app testing. You can leverage frameworks like Appium, which extends Selenium to support mobile automation. Appium allows you to automate testing on mobile emulators and real devices, enabling you to write cross-platform tests that can run on iOS and Android platforms.
Recent updates and new features: Selenium is a dynamic and evolving framework, and new features are introduced regularly to enhance test automation capabilities. It’s recommended to stay updated with the latest releases and documentation of Selenium Python bindings to leverage the new features and improvements. Recent updates might include enhancements in handling web elements, improved browser compatibility, support for new browser versions, and optimizations for test execution speed.
By exploring these advanced techniques and features with Selenium Python bindings, you can handle complex test scenarios, perform sophisticated interactions, and extend your automation efforts to mobile app testing. Keep an eye on the Selenium community and documentation to stay informed about the latest updates and advancements in Selenium Python bindings, ensuring that you make the most of this powerful automation tool.
Conclusion
Selenium Python bindings are one of the easiest ways to integrate Python with Selenium. The binding helps us in creating easy-to-use APIs to write the functional/acceptance tests for Selenium Webdriver. This way we can easily write test scripts on Python to test the various functionalities and browsers behaviours on the Selenium WebDriver. In the blog we have seen the example of using the Locate Elements method. However, there are many more methods to test such as the wait, navigation, page objects functionality in the browsers. Selenium Python Binding makes a power-pact duo in accelerating our web app and website testing efforts. We have attached a helpful whitepaper to learn more about automation using selenium, feel free to download your free copy.
